経緯
個人的にはどうしても後回しにされがちなテストコードについて、少しでも精神的な障壁を下げるべく Jest を軽く触ってみることにしました。
PHPUnit についてはPHPUnitとXdebugでコードカバレッジの出力を試すで触ったことがあるので、ユニットテストの雰囲気は掴んでいます。
そこで今回は JS 側を……ということで Jest を触ってみることにします。
今回は本当に最初の最初だけ。基本的にはJestで始める! ユニットテスト – 環境の準備とテストの実行 | CodeGridの記事の通りに進めます。
準備
package.json
{
"name": "finality_jest",
"version": "0.0.1",
"description": "jestのテスト",
"main": "sum.js",
"scripts": {
"test": "jest",
"test:coverage": "jest --coverage"
},
"author": "",
"license": "ISC",
"devDependencies": {
"jest": "^26.6.3"
}
}
npm init
した後 yarn add --dev jest
で Jest を追加。 npm scripts にテスト実行とカバレッジ出力のスクリプトを追記。
sum.js
const sum = (a, b) => {
return a + b;
};
module.exports = sum;
ほぼ記事のママです。
jest.config.js
module.exports = {
coverageDirectory: 'coverage'
};
現状では有効なオプションを指定していませんが、 coverages
に変更してカバレッジ出力されるディレクトリが変化したことは確認できたので、後はテストケースに併せてこの辺(英: Configuring Jest · Jest, 日: Configuring Jest · Jest)のオプションをカスタマイズしていけば良いのかな、と思います。
test/sum.test.js
const sum = require('../sum');
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
こちらもほぼママ。実際に使用する場合はテストコードが増えていくと思われるので予めディレクトリに入れておく方向性で考えました。
その場合、ファイル名末尾を .test.js
にする必要はないのですが、実際のコードと同じ名前にすると、今回は sum.js
と __test__/sum.js
の区別がエディタ上で付きづらいので、結局 sum.test.js
と末尾に .test.js
を付ける方向の方が良いのかな、と感じました。
ディレクトリ構造
以上を踏まえてディレクトリ構造は以下のようになりました。
/
├ __test__/
│ └ sum.test.js
├ .gitignore
├ jest.config.js
├ package.json
└ sum.js
実行結果
これでテストを実行。
> yarn test:coverage
$ jest --coverage
PASS __tests__/sum.test.js
√ adds 1 + 2 to equal 3 (3 ms)
----------|---------|----------|---------|---------|-------------------
File | % Stmts | % Branch | % Funcs | % Lines | Uncovered Line #s
----------|---------|----------|---------|---------|-------------------
All files | 100 | 100 | 100 | 100 |
sum.js | 100 | 100 | 100 | 100 |
----------|---------|----------|---------|---------|-------------------
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Snapshots: 0 total
Time: 4.061 s
Ran all test suites.
Done in 6.86s.
OKですね。
試しに sum.js
を以下のように変更します。
const sum = (a, b) => {
return a - b;
};
module.exports = sum;
加算ではなく減算にしました。これで再度テスト実行。
> yarn test:coverage
yarn run v1.22.4
$ jest --coverage
FAIL __tests__/sum.test.js
× adds 1 + 2 to equal 3 (6 ms)
● adds 1 + 2 to equal 3
expect(received).toBe(expected) // Object.is equality
Expected: 3
Received: -1
2 |
3 | test('adds 1 + 2 to equal 3', () => {
> 4 | expect(sum(1, 2)).toBe(3);
| ^
5 | });
6 |
at Object.<anonymous> (__tests__/sum.test.js:4:23)
----------|---------|----------|---------|---------|-------------------
File | % Stmts | % Branch | % Funcs | % Lines | Uncovered Line #s
----------|---------|----------|---------|---------|-------------------
All files | 100 | 100 | 100 | 100 |
sum.js | 100 | 100 | 100 | 100 |
----------|---------|----------|---------|---------|-------------------
Test Suites: 1 failed, 1 total
Tests: 1 failed, 1 total
Snapshots: 0 total
Time: 4.363 s
Ran all test suites.
error Command failed with exit code 1.
怒られますね。OKです。
元に戻して再度実行。
今度は生成された coverage
ディレクトリを辿ります。 coverage/lcov-report/
を開くと以下のようにカバレッジが出力されていました。
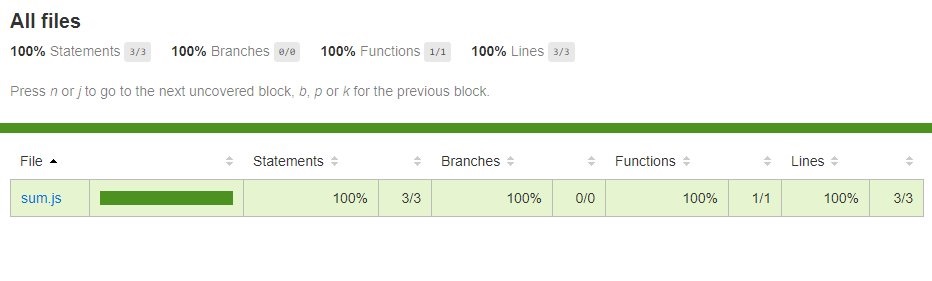
参考記事の通り、パッケージ一つでここまでできると助かりますね。
備考
一応リポジトリを貼っておきます。